API Gateway Design with OAuth2
For example, UBER may expose API's that allow a user to request a ride, cancel ride, view payment history etc. GROUPON may expose API's to update user profile, list user related promotions etc.
A micro-services architecture based application may constitute of a large number of independent services and it may not be realistic to duplicate and implement the API security logic at every layer or service. Its best to centralize the security layer so as to make the API governance easy.
In this article I will describe a simple design for an API gateway which can be used to authorized and control access to underlying API's. The API gateway is a simple PROXY service to underlying API's but with an added Authorization layer.
User Authentication
The first step to gain access to API's are to establish the user identity. A typical flow is shown below: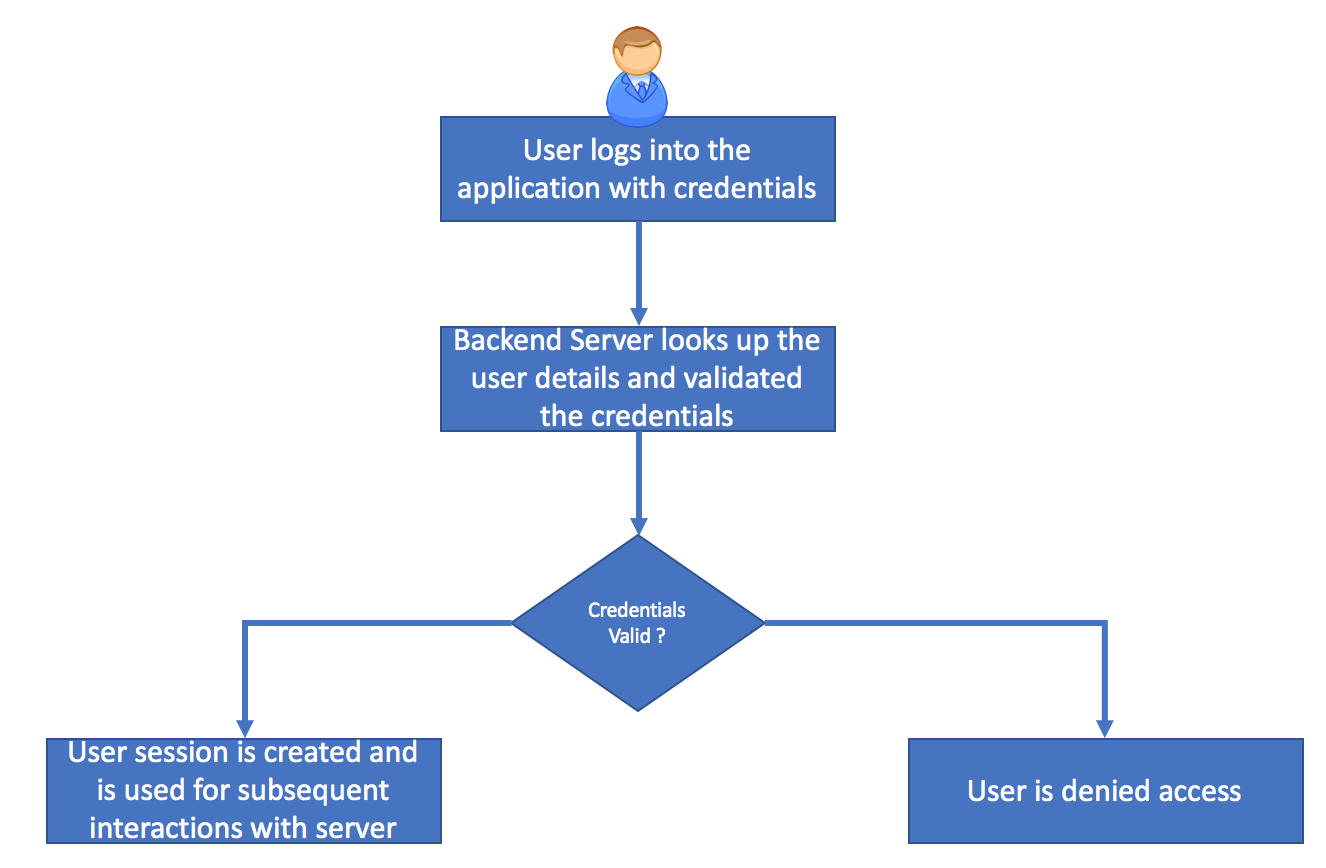
The idea is to delegate the user authentication to a dedicated service which looks up user details from the data store, validates it and grants permissions/access. This service will use OAuth2 concepts. OAuth2 is a standardized protocol for authorization. It follows a simple concept of creating a set of token for each representing an authorized user and associating permissions/grants with those tokens.
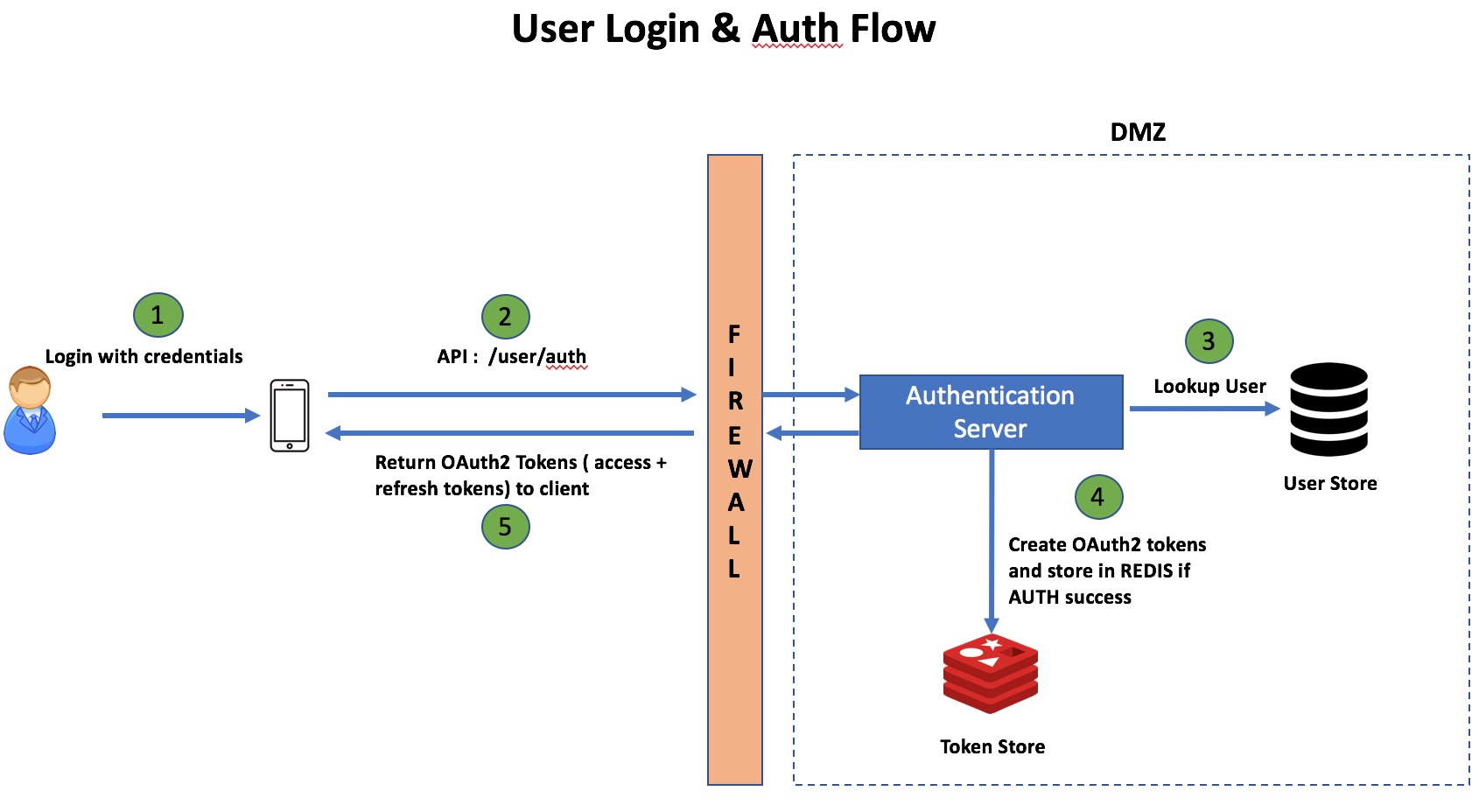
Why use REDIS as Token Store ?
- 1. Allows super fast reads and large number of read-write operations per second.
- 2. A TTL can be set on each token to auto expire. Save the trouble of building a separate service to clean up old tokens.
- 3. REDIS cluster can be scaled horizontally based on login traffic
API Gateway
The API gateway is the only service that will be exposed outside the firewall. It acts as a proxy to underlying API's in the microservice and does a quick authorization and look up of user information from REDIS. The user information especially the userId is used to invoke the underlying APIs.
The gateway also does the job of passing down other user related information like grants to underlying service to help customize responses based on what the user is allowed to access.
This layer can be easily scaled to accomodate increased login traffic and also quickly blocks any DDOS attacks without consuming or affecting resources on the underlying data stores or other microservices.
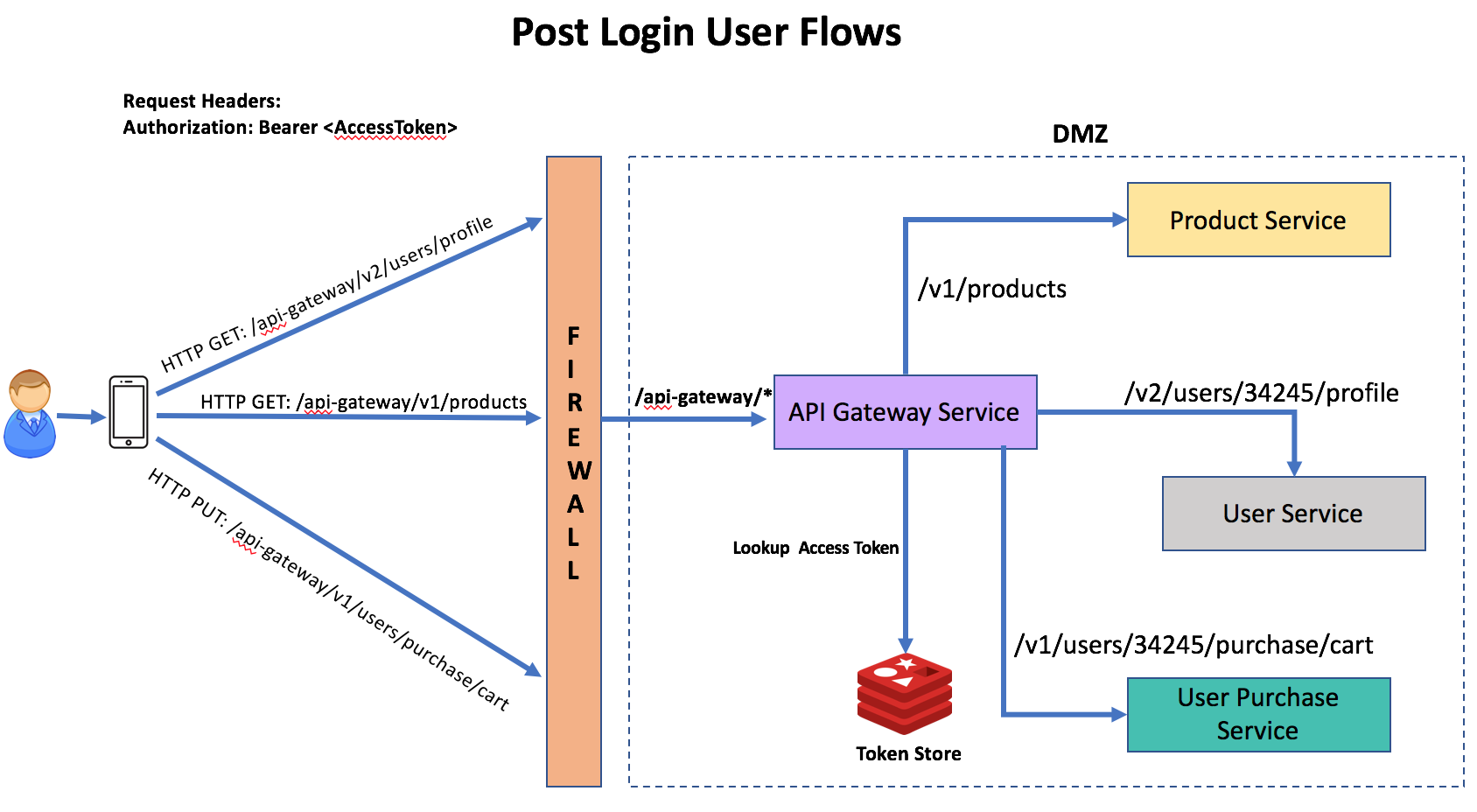
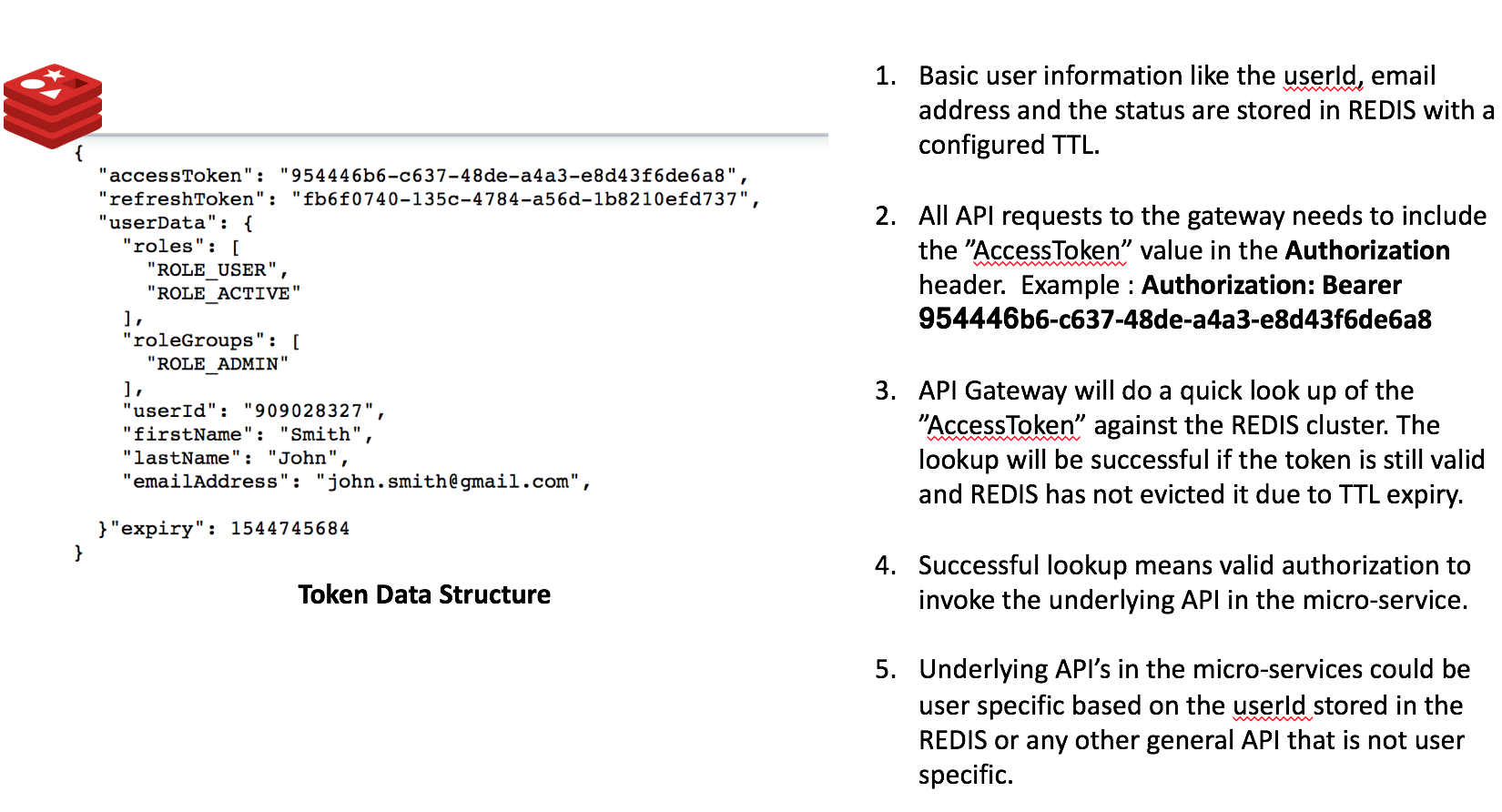
Implementation Notes
- 1. The API gateway layer can be implemented as a simple JAVA application with RxJava to do non-blocking API calls and stream the request/response data between client and server.
- 2. Jedis is a good java library for interfacing with REDIS
- 3. Spring OAuth library provides implementations that can be overridden to hook into different user stores and add customized logic for user retrival, validation and token metadata.